At wercker we have added build and deployment status output in the cctray.xml format, an RSS-like continuous integration server status feed, which was originally developed for CruiseControl.net. In this post I would like to share the details and how I leverage this to get notified on important wercker status changes via CCMenu.
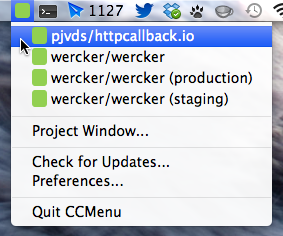
Configuration
Wercker offers two endpoints per project that serve status information in the cctray.xml format. One for the build status, and one for the deployment status.
Buids status feed
The feed url that contains the build status from an project is:
https://app.wercker.com/api/v2/applications/{PROJECT-ID}/cc/build
You need to replace {PROJECT-ID}
with the project id of the project you want to monitor.
Deploy status feed
The feed url that contains the deployment status of a project's deploy target is:
https://app.wercker.com/api/v2/applications/{PROJECT-ID}/cc/deploytargets/{DEPLOY-TARGET-NAME}
You need to replace {PROJECT-ID}
with the project id of the project you want to monitor and {DEPLOY-TARGET-NAME}
with the name of the deploy target. Read more about deploy targets on ou dev center
Tools
Here is a list of tools that allow you to monitor cctray.xml feeds and that can be used to monitor your build and deployment statuses of your wercker projects.
Using CCMenu
There are a lot of tools that support the cc status format. As I'm mostly working on a Mac I decided to use CCMenu. It allows you to monitor multiple projects at the same time, and identifies the overall build status with just a glance at the menu bar.
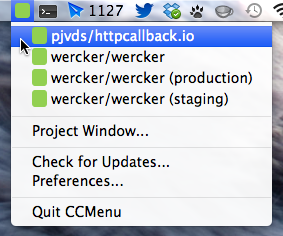
Download and install the latest version of ccmenu from the CCMenu project.
Finding your project id
Navigate to your project page at app.wercker.com and copy your project id from the url.
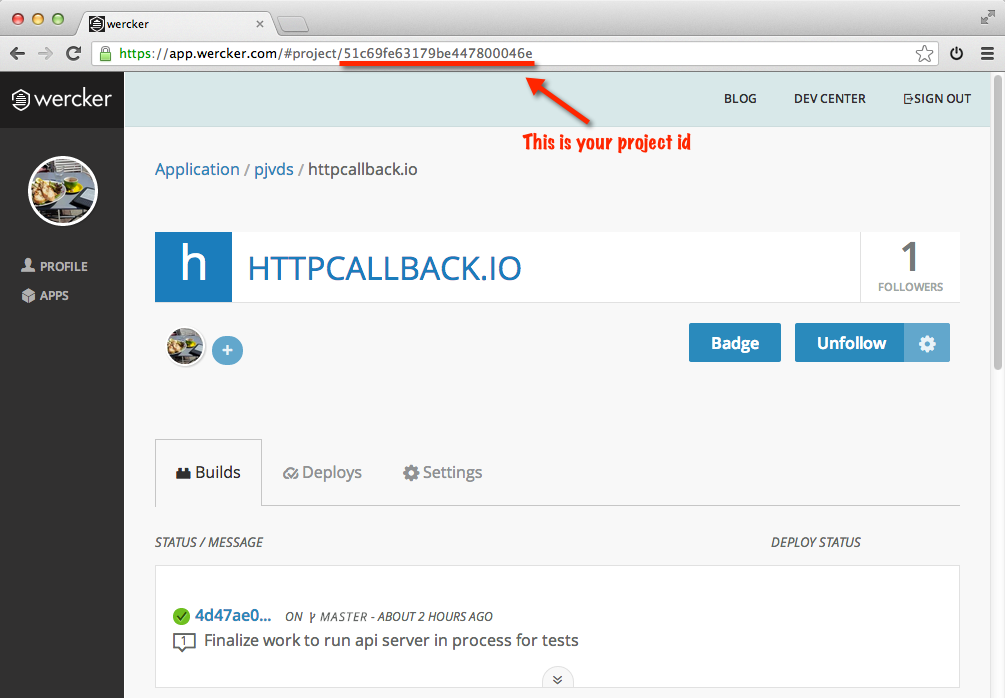
Add builds to CCMenu
Open CCMenu by clicking on the icon in the top menu bar and open preferences.
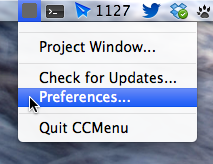
Enter feed details
Click on the plus sign in the preference window and enter your project cc
feed url. It is important to follow the following steps closely because CCMenu was acting a bit weird when I entered my details.
- Enter your feed url
- Click
Use URL as entered above
option
- Enter your wercker credentials
- Check
The server requires authentication
- Click
Continue
- See the status of your project in your menu bar!
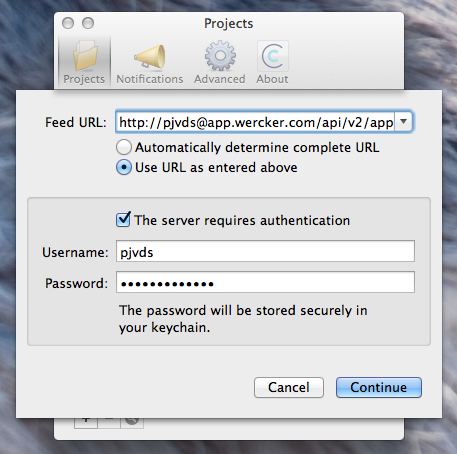
Other feeds
You can repeat the previous steps for other feeds as well.
Notification center
CCMenu supports Mac's Notification Center
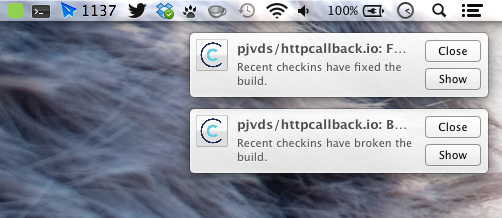
Final result
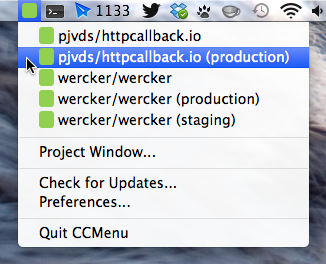
Earn some stickers
Let me know about the applications you build with wercker. Don't forget to tweet out a screenshot of your first green build with #wercker and I'll send you some @wercker stickers.